This project was conducted as part of my internship with the Virtual Human Interaction Lab under the VRITS (Virtual Reality Intensive Training Seminar).
After developing over 100 Virtual Reality Worlds Using Engage software alongside my VRITS team in Fall of 2021 as part of a Cutting-Edge Class taught entirely in VR, I was tasked with gathering dimensionality data on the size of Each of the worlds. Our team was aiming to see if there was any relationship between the size and type (indoor vs. Outdoor) of a Virtual World and the type/amount of communication and interaction among the students that were in that world.
Data To Work With
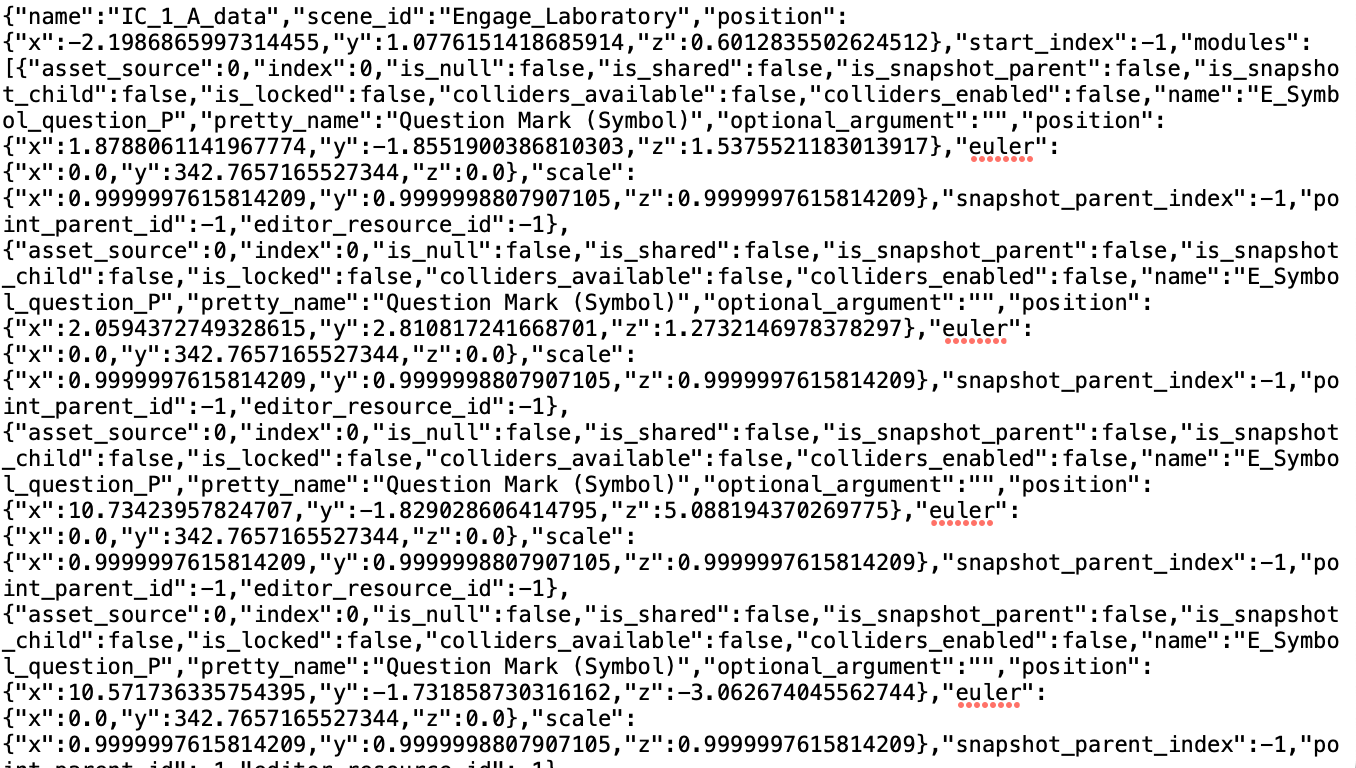
ABOVE IS A TEXT FILE REPRESENTING THE POSITIONAL INFORMATION of all of the objects in a virtual environment. For each of the virtual worlds, we have a file At first glance, it appears as a jumbled file of untraceable information. However, using clever regular expressions to extract specific information from these files will allow us to acquire the data we need in order to compute the dimensionality data.
Prior to scripting the program to acquiring such information, we first needed specific data to extract that will enable us to calculate the dimensionality data. Since the files for each virtual world track the position of each object, I went in to each world and placed arbitrary objects at the perimeter of the virtual worlds as well as the ground and ceiling/sky levels. By doing this, these objects would appear within their respective object files and thus would be accessible to my program.
first, we must Develop a Proper Regex to extract the Object Locations. Below is how our sample object, used as a place marker (i.e., a question mark object), appears in the text file:
"Question Mark (Symbol)","optional_argument":"","position":{"x":1.8788061141967774,"y":-1.8551900386810303,"z":1.5375521183013917}
And the following is the Regular expression that we can use to extract information of this type within a larger text file as shown above (syntax Based on the PyThon Regular expression library):
"(?:Question Mark \(Symbol\)\"\,\"optional_argument\"\:\"\"\,\"position\"\:\{)(?:\"x\"\:)(-?[0-9]+\.[0-9]+)\,(?:\"y\"\:)(-?[0-9]+\.[0-9]+)\,(?:\"z\"\:)(-?[0-9]+\.[0-9]+)\}"
The following code snipbit loops through all of the text files and uses the regex above to extract the locations of each of the place markers. To calculate the height of the world, we simply take the marker at the highest point and the ground marker and take their y-coordinate difference.
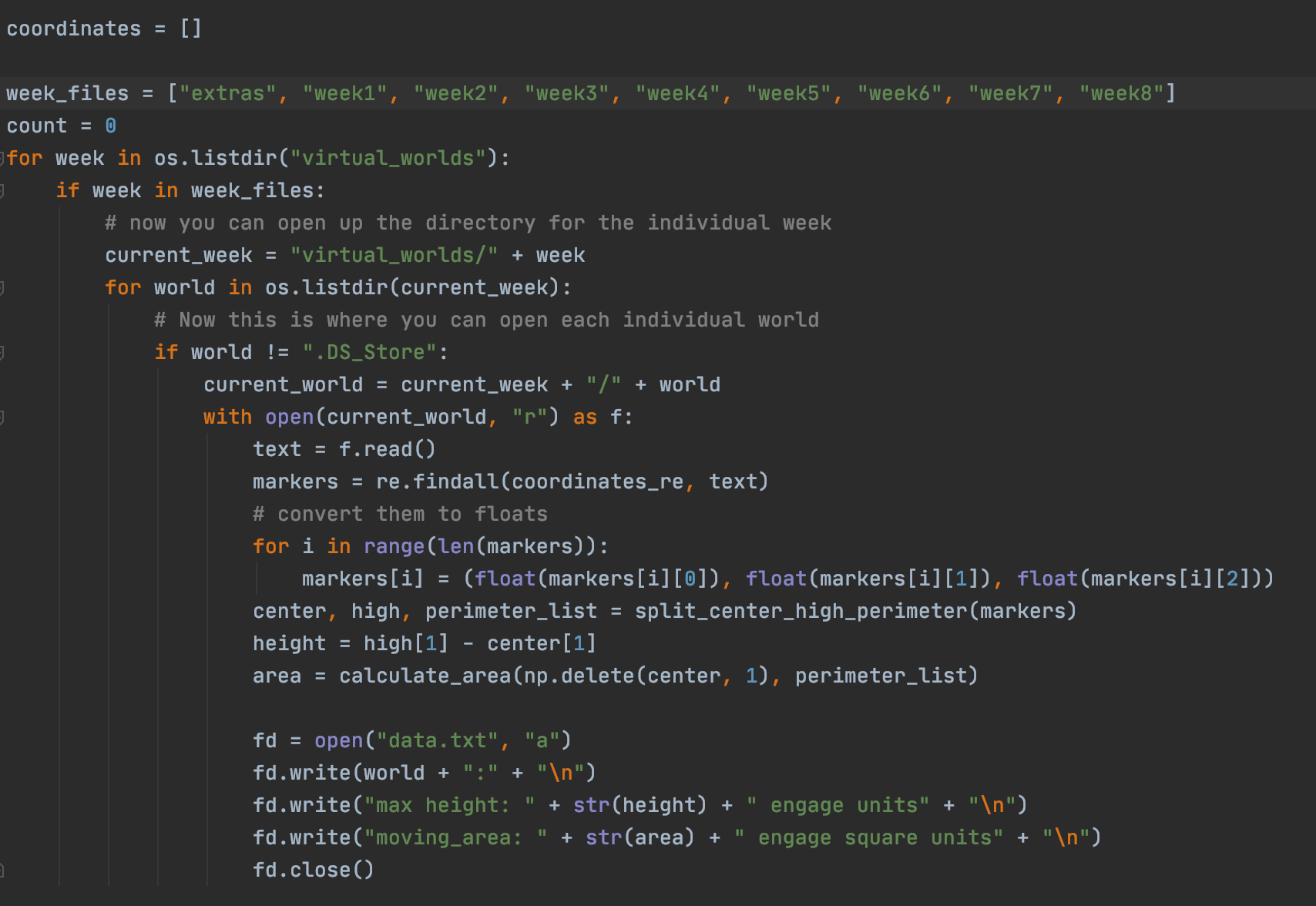
Next, we will use the positions of our place markers to calculate the Ground area or movement space.
To calculate the area, we must first find the center marker so we know which marker to pivot around when triangulating the Area. To do this, we first eliminate the highest Marker (which was used to calculate the height of the world) and then find the average of all the remaining points. From here, we know that the center pivot marker is the marker that is closest to this average center point. We can find this marker by using the scipy spatial distance library and specifically the cdist function on all the points, as shown Below.
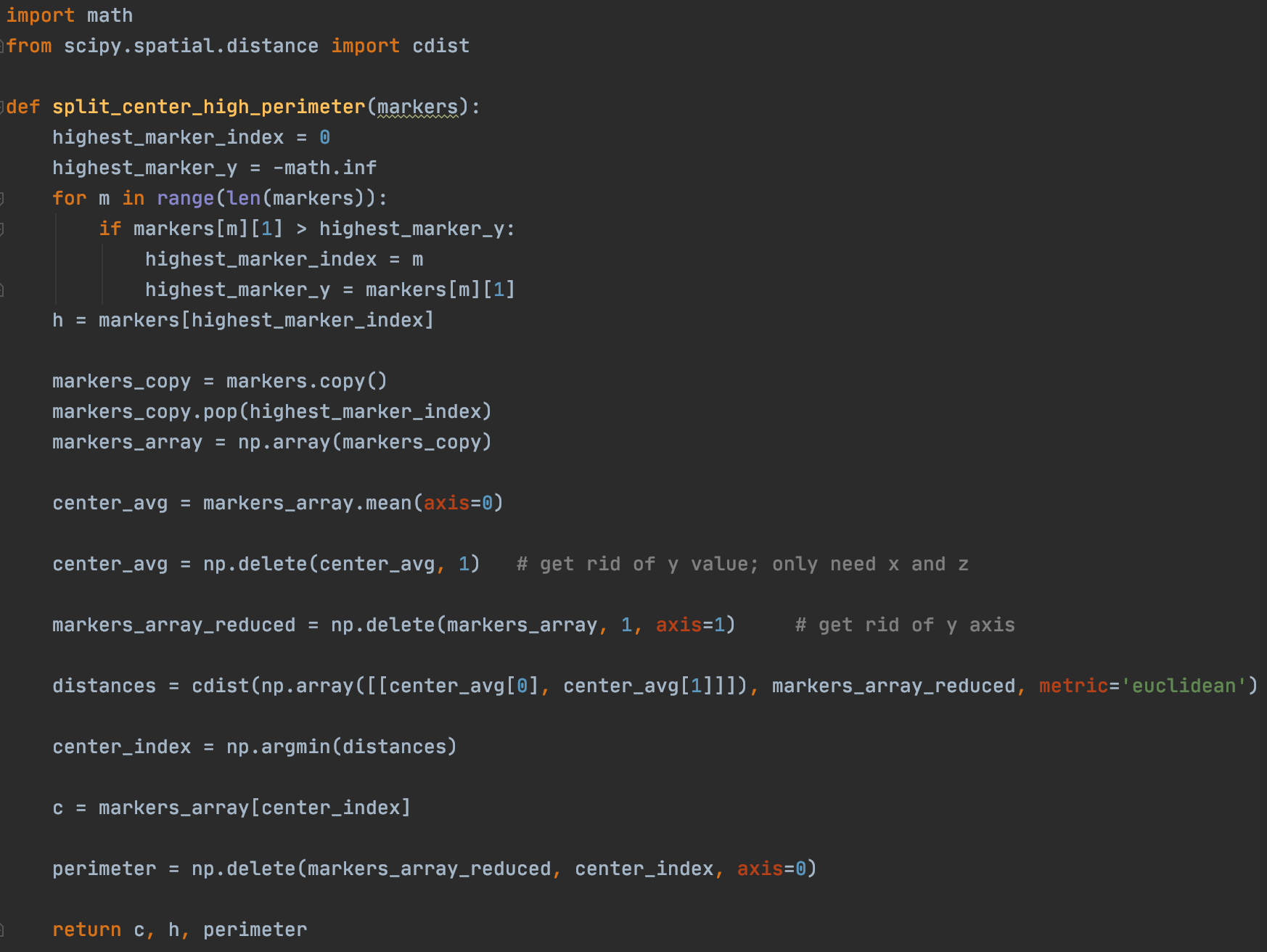
Next, we must find the order in which to use points to triangulate the region, and we can do this by using vectors to find radian degrees between points, and then sorting the vectors by their radian degrees. This process is shown in the first for loop in the function below.
We could then triangulate the area. If we only have a perimeter of 4 points, it would actually be easier to simply split the region up into two triangles and sum their areas, which is what the first If-Block does. For cases where the perimeter is more complex (more perimeter points), We iterate through the sorted vectors and create triangles as we go, calculating their area, and then adding it to the total.
After these steps we would have the height of the world as well as the area of the player movement space, exactly what we need to conduct our analysis.
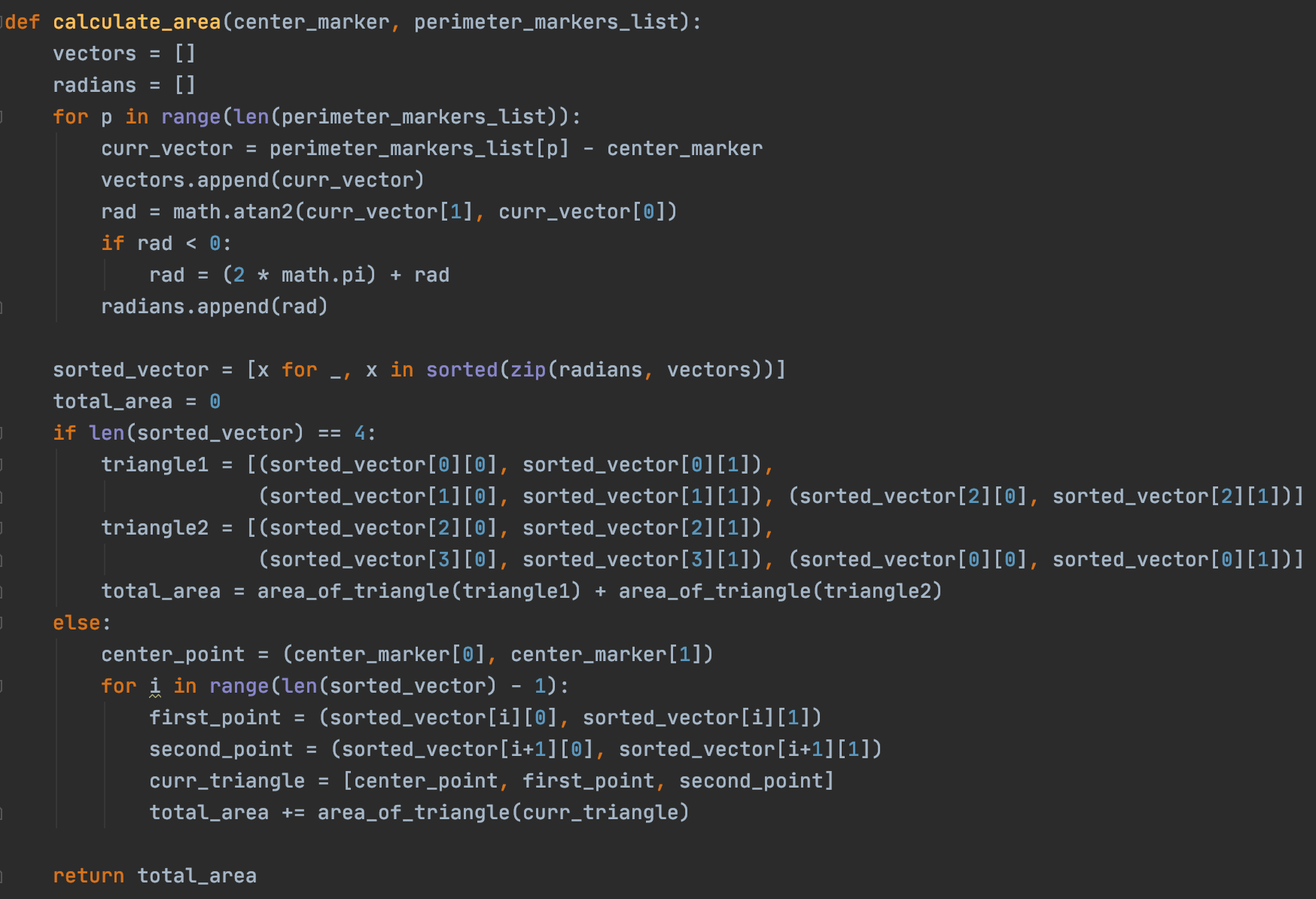
Additional Helper functions
Below is a helper function to calculate the area of a triangle. In our situation, it is easiest to calculate the area using Heron's formula.
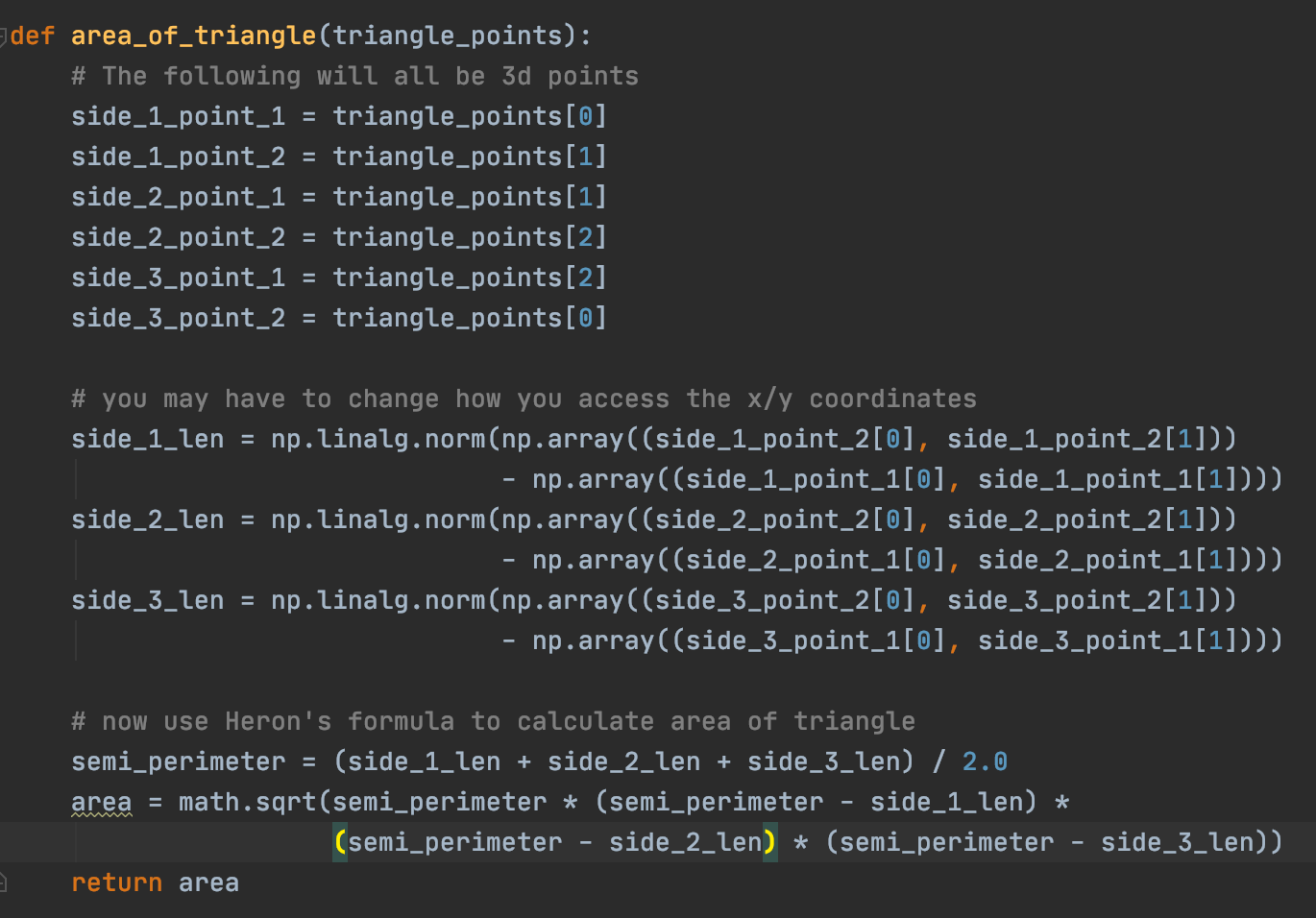
Output
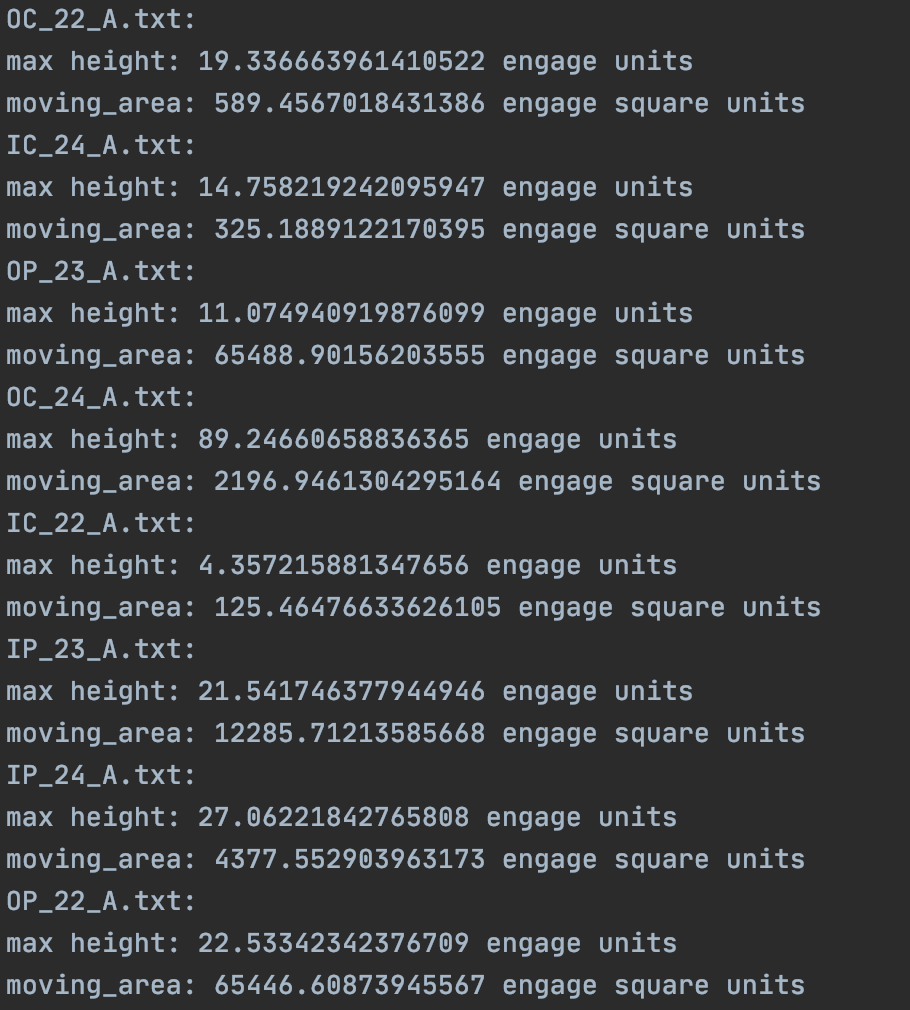
Terms such as IC_22_A and IP_23_A are just identifiable names for each of the Virtual worlds for purposes of data recording and organization.
Additional Skills
This project involved porting virtual environments from the OCulus Quest Headset and extracting its corresponding json file in order to work with it in this program.
A Note from Umar
I avoid simply placing my computer science projects on GitHub. I personally believe that GitHub doesn't do as well of a job as showcasing the process of constructing a computer science project as a portfolio would. Therefore, I aim to display all my personal and internship projects on this site, showing not only my code but also my thought process in constructing the program.